결과 화면
Plus 버튼을 누르면 숫자가 1 증가하고, Minus 버튼을 누르면 숫자가 1 감소한다.
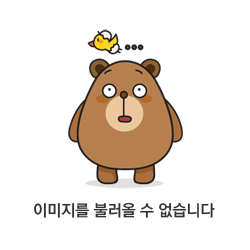
1. 바닐라 자바스크립트로 카운터 만들기
- index.html
<body>
<button id="minus">Minus</button>
<span id="number"></span>
<button id="plus">Plus</button>
</body>
- index.js
// html 태그 선택
const plus = document.getElementById('plus')
const minus = document.getElementById('minus')
const number = document.getElementById('number')
// 초기값
let count = 0
// html 화면에 초기값 count 보여주기
number.innerText = count
// count가 변할 때 마다 화면에 보여주기
const updateText = () => {
number.innerText = count
}
// count +1
const handlePlus = () => {
count++
updateText()
}
// count -1
const handleMinus = () => {
count--
updateText()
}
// 버튼 클릭 이벤트
plus.addEventListener('click', handlePlus)
minus.addEventListener('click', handleMinus)
※ 리덕스 기본 개념
- store
data를 저장하는 곳이다.
import { createStore } from 'redux'
const store = createStore(reducer)
- state(상태)
변경이 필요한 data이다.
- reducre
state를 변경하는 function(함수)이다.
reducer의 return 값이 state가 된다.
const reducer = (state, action) => {}
- action
reducer의 두번째 파라미터 값이다.
action을 통해서만 state를 변경할 수 있다.
action은 object(객체)타입만 가능하고, 그 key 값은 type만 가능하다. (action.type)
공식문서에 따르면 if문 보다는 switch문이랑 더 자주 사용된다.
- dispatch
reducer에 action을 보내는 방법이다.
dispatch를 통해서만 action을 실행시킬 수 있다.
현재 이벤트를 발생시키는 함수라고 볼 수 있다.
store.dispatch({type: 액션이름})
- subscribe
store 안에 있는 변화를 감지한다.
store.subscribe(함수호출)
2. 리덕스로 카운터 만들기
yarn add redux 또는 npm install redux
- index.html
<body>
<button id="plus">Plus</button>
<span id="number"></span>
<button id="minus">Minus</button>
</body>
- index.js
import { createStore } from 'redux'
// html 태그 선택
const plus = document.getElementById('plus')
const minus = document.getElementById('minus')
const number = document.getElementById('number')
// 초기값
number.innerText = 0
// string을 직접 사용하기 보다는 변수로 만들어서 사용하는 것이 오타 등의 에러를 발견할 수 있어 효율적이다.
const ADD = 'ADD'
const MINUS = 'MINUS'
// 2. reducer(변수 이름은 자유)를 만든다.
const reduceCountModifier = (count = 0, action) => {
// 3. action을 만든다.
switch (action.type) {
case ADD:
return count + 1
case MINUS:
return count -1
default:
return count
}
}
// 1. store를 만든다.
const countStore = createStore(reduceCountModifier)
// store 안에 있는 state를 가지고 온다.
const onChange = () => {
number.innerText = countStore.getState()
}
// store 안에 있는 state의 변화를 감지한다.
countStore.subscribe(onChange)
// 4. dispatch를 만든다.
const handlePlus = () => {
countStore.dispatch({type: ADD})
}
const handleMinus = () => {
countStore.dispatch({type: MINUS})
}
// 클릭 이벤트
plus.addEventListener('click', handlePlus)
minus.addEventListener('click', handleMinus)
'React > React with redux' 카테고리의 다른 글
[리액트][리덕스] #3.2 React Redux (To Do List 삭제하기) - 초보자를 위한 리덕스 101 (0) | 2023.04.28 |
---|---|
[리액트][리덕스] #3.1 React Redux (To Do List 추가하기) - 초보자를 위한 리덕스 101 (0) | 2023.04.28 |
[리액트][리덕스] #3.0 React Redux (To Do List 세팅하기) - 초보자를 위한 리덕스 101 (0) | 2023.04.28 |
[리덕스] #2 Pure Redex: To Do List - 초보자를 위한 리덕스 101 (0) | 2023.04.27 |
[리덕스] #0 Redux Introduction - 초보자를 위한 리덕스 101 (0) | 2023.04.20 |